Introduction
Accept EBT payments in your Android mobile app
Looking for the Forage mobile app?
Get instant EBT balance checks and access to exclusive grocery deals—download Forage on iOS or Android and start saving today.
You can use the Forage Android SDK to process EBT payments in your Android app.
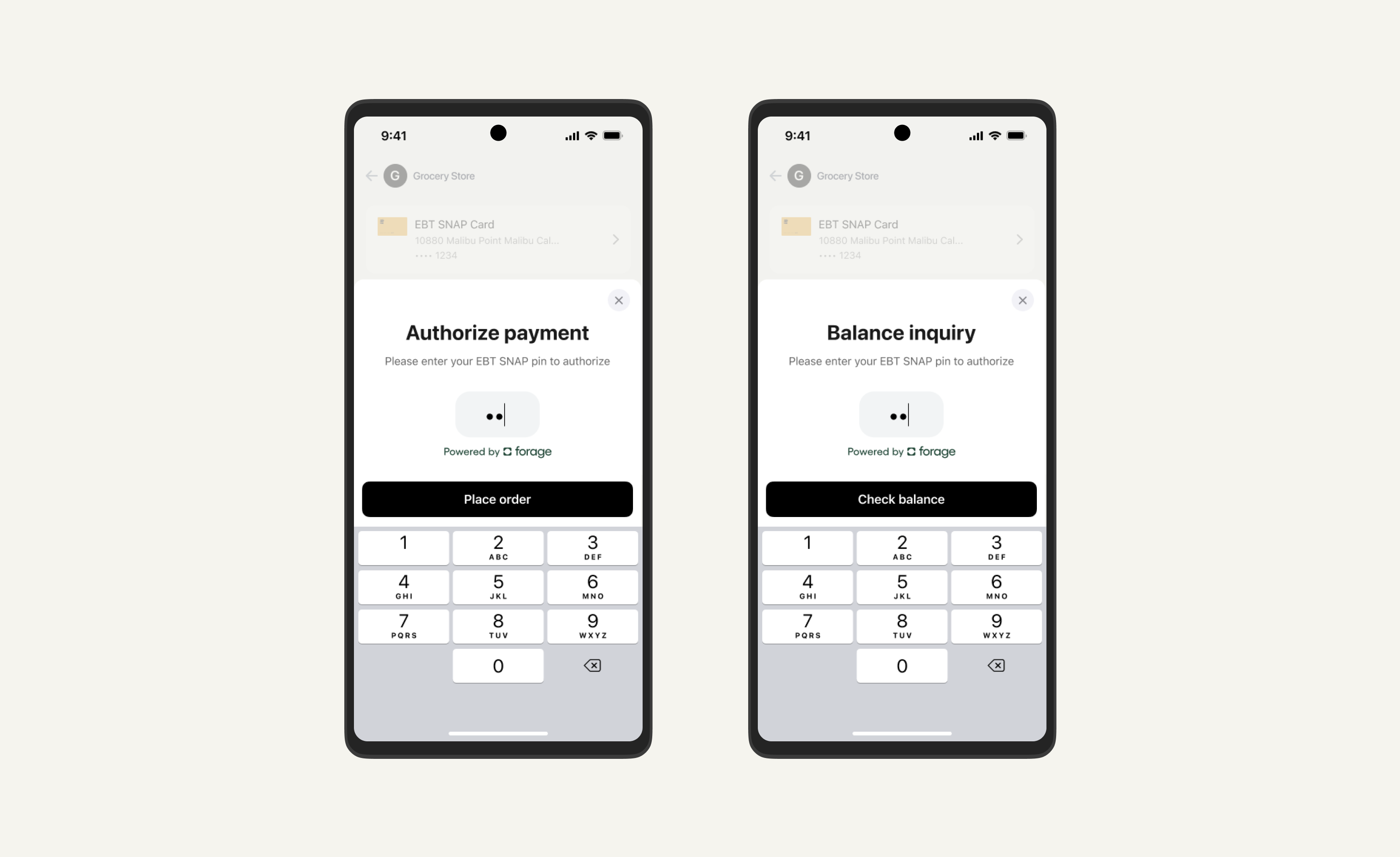
Features
- Native UI component for collecting and tokenizing a customer’s EBT Card number (also called the card PAN)
- Native UI component for collecting a customer’s EBT PIN
- Built-in EBT Card number validation
- Control over the styling of a native EBT checkout experience
- API methods that collect and tokenize an EBT Card number, perform a balance check, and capture a payment
Requirements
Before you can use the Forage Android SDK, make sure that you have:
- Met all of the prerequisites for working with Forage.
- Signed up for an account. Contact us if you don’t yet have one.
- Built your app for Android API Level 23 (Android 6 Marshmallow) or newer.
For more information, see:
Starter code
After installing the library, include either or both of the UI components in your application’s views.
For example, the following snippet adds the EBT Card number element ForagePanEditText
:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.joinforage.forage.android.ecom.ui.element.ForagePANEditText
android:id="@+id/foragePanEditText"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
android:textColor="?android:attr/textColor"
android:textSize="?android:attr/textSize"
app:panBoxStrokeColor="?attr/panBoxStrokeColor"
app:panBoxStrokeWidthFocused="?attr/panBoxStrokeWidthFocused"
app:panBoxStrokeWidth="?attr/panBoxStrokeWidth"
app:cornerRadius="?attr/cornerRadius"
app:textInputLayoutStyle="?attr/textInputLayoutStyle" />
</androidx.constraintlayout.widget.ConstraintLayout>
Then, reference the element in response to a user action. The example below tokenizes the EBT Card number and could be included within an onSubmit
function:
// TokenizeViewModel.kt
@HiltViewModel
class TokenizeViewModel @Inject constructor(
savedStateHandle: SavedStateHandle,
private val moshi: Moshi
) : ViewModel() {
private val args = TokenizeFragmentArgs.fromSavedStateHandle(savedStateHandle)
// internal so that TokenizeFragment can access these values
val merchantID = args.merchantAccount
val sessionToken = args.sessionToken
fun tokenizeEBTCard(foragePanEditText: ForagePANEditText) = viewModelScope.launch {
_isLoading.value = true
val response = ForageSDK().tokenizeEBTCard(
TokenizeEBTCardParams(
foragePANEditText = foragePanEditText,
reusable = true,
// NOTE: The following line is for testing purposes only and should not be used in production.
// Please replace this line with a real hashed customer ID value.
customerId = UUID.randomUUID().toString(),
)
)
when (response) {
is ForageApiResponse.Success -> {
val adapter: JsonAdapter<PaymentMethod> = moshi.adapter(PaymentMethod::class.java)
val paymentMethod = adapter.fromJson(response.data)
// (optional) do something with the ref
saveToPaymentMethodRefToMyAPI(paymentMethod.ref)
}
is ForageApiResponse.Failure -> {
_error.value = response.message
}
}
_isLoading.value = false
}
}
Next steps
- Check out the forage-android-sdk GitHub repository for full installation instructions and a demo app
Updated 5 days ago