Introduction
Add EBT payments to your iOS app
Looking for the Forage mobile app?
Get instant EBT balance checks and access to exclusive grocery deals—download Forage on iOS or Android and start saving today.
You can use the Forage iOS SDK to process EBT payments in your iOS app.
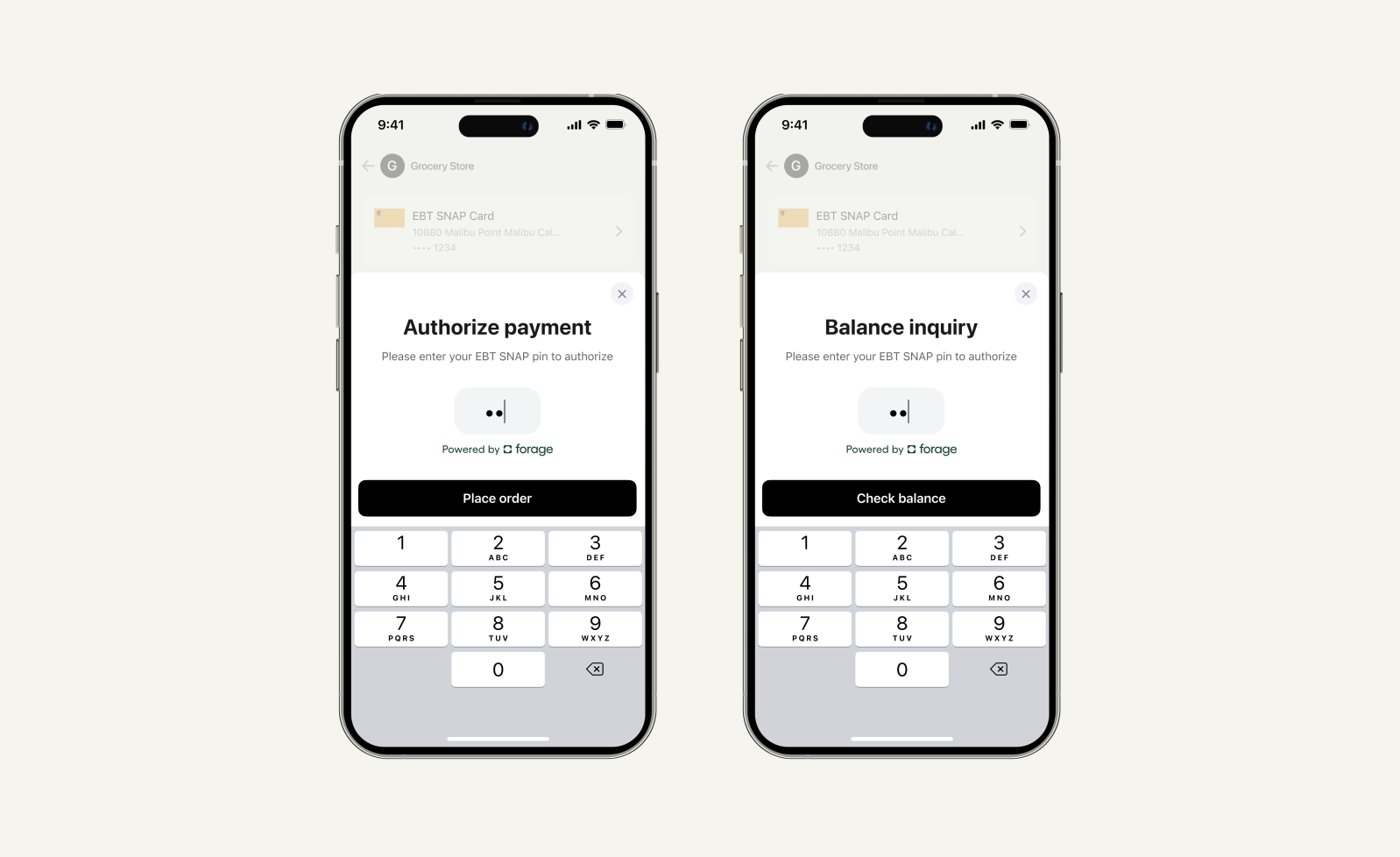
Features
- Native UI component for collecting and tokenizing a customer’s EBT Card number (also called the card PAN)
- Native UI component for collecting a customer’s EBT PIN
- Built-in EBT Card number validation
- Control over the styling of a native EBT checkout experience
- API methods that collect and tokenize an EBT Card number, perform a balance check, and capture a payment
Requirements
Before you can use the Forage iOS SDK, make sure that you have:
- Met all prerequisites for working with Forage
- Signed up for an account. Contact us if you don’t yet have one.
- Your app is built on the following:
- iOS 13+
- Swift 5
For more information, see:
Starter code
After setting up your development environment and importing and initializing the SDK, include either or both of the UI components in your application’s views.
For example, the following snippet adds the EBT Card number element ForagePANTextField
:
private let foragePanTextField: ForagePANTextField = {
let tf = ForagePANTextField()
tf.borderColor = .black
tf.borderWidth = 3
tf.clearButtonMode = .whileEditing
tf.cornerRadius = 4
tf.font = .systemFont(ofSize: 22)
tf.placeholder = "EBT Card Number"
return tf
}()
Then, call the relevant method. The below snippet calls the method to tokenize an EBT PAN:
// Usage
ForageSDK.shared.tokenizeEBTCard(
foragePanTextField: foragePanTextField,
// NOTE: The following line is for testing purposes only and should not be used in production.
// Please replace this line with a real hashed customer ID value.
customerID: UUID.init().uuidString,
reusable: true
) { result in
// Handle result and error here
}
Next steps
- Check out the forage-ios-sdk GitHub repository for full installation instructions and a demo app
Updated 25 days ago