Receipts and confirmation screens
Learn about FNS requirements for transaction records and how to extract the data
A confirmation screen is a display within a merchant’s website or app that immediately alerts a customer to the status of their purchase, refund, or failed transaction attempt.
A receipt is a record of a customer’s transaction. Receipts give customers proof of purchase, refund, or failed transaction attempt.
FNS requires that receipts and confirmation screens for EBT SNAP online include specific information. This guide details what that information is and where to find it. Read on for an introduction to the Forage receipt
object, or skip to FNS requirements for order confirmation screens and receipts.
Jump To FNS Requirements
To skip the technical implementation details, head to FNS requirements.
Forage receipt
object
receipt
object{
"ref_number": "591bbfe14a",
"is_voided": false,
"snap_amount": "20.00",
"ebt_cash_amount": "0.00",
"other_amount": "0.00",
"sales_tax_applied": "0.00",
"balance": {
"id": 29103,
"snap": "80.00",
"non_snap": "100.00",
"updated": "2023-11-07T05:17:22.824579-08:00"
},
"last_4": "5455",
"message": "Approved",
"transaction_type": "Order",
"created": "2023-11-07T05:17:23.122849-08:00"
}
The Forage receipt
object includes most of the information that FNS requires merchants to display to EBT cardholders. Forage returns a receipt
in response to calls to specific Payments API endpoints and SDK methods.
Receipt Data Availability
The
receipt
value remains null until the associated transaction is captured, except in the case of refunds. Receipt data for a refund becomes available as soon as it is created.A payment is captured using the SDK's submit function, while an order is captured through the Forage Checkout UI.
For more details, please refer to How to Retrieve Receipt Data.
receipt
properties
receipt
propertiesProperty | Type | Description |
---|---|---|
ref_number | string | A unique reference hash for the Forage Order , Payment , or Refund associated with this receipt. |
is_voided | boolean | Whether the transaction associated with this receipt has been voided. If false , then the transaction is proceeding as expected; if true , then the transaction is to be reversed. |
snap_amount | string | The USD amount charged/refunded to the SNAP balance of the EBT Card, represented as a numeric string. |
ebt_cash_amount | string | The USD amount charged/refunded to the EBT Cash balance of the EBT Card, represented as a numeric string. |
other_amount | string | The USD amount charged/refunded to any payment method that is not an EBT Card, represented as a numeric string. |
sales_tax_applied | string | The USD amount of taxes charged to the customer’s non-EBT payment instrument, represented as a numeric string. |
balance | object | Refer to balance for details. |
last_4 | string | The last four digits of the EBT Card number. |
message | string | A message from the EBT payment network. |
transaction_type | string | A constant string that is used to identify the transaction type associated with the receipt. One of: - Order - Payment - Refund |
created | ISO 8601 date-time string | A timestamp of when the transaction object was created. |
balance
properties
balance
propertiesProperty | Type | Description |
---|---|---|
snap | string | The available SNAP balance in USD on the customer’s EBT Card, represented as a numeric string. |
non_snap | string | The available EBT Cash balance in USD on the EBT Card, represented as a numeric string. |
updated | ISO 8601 date-time string | A timestamp of when the funds in the account last changed. |
How to retrieve receipt
data
receipt
dataReceipt data populates when a transaction’s status
is succeeded
.
For orders, pass the ref
returned in the response that created the order's parent Session
in periodic GET requests to /orders/{order_ref}/
until the status
of the Order
is succeeded
.
For payments, pass the ref
returned in the response to create the Payment
in periodic GET requests to /payments/{payment_ref}/
until the status
of the Payment
is succeeded
.
Endpoints that return a receipt
receipt
- Sessions
- Create a Fully Hosted Session: POST
/sessions/
- Create a Custom Payment Capture Session: POST
/capture_sessions/
- Create a Fully Hosted Session: POST
- Orders
- Retrieve an Order: GET
/orders/{order_ref}/
- Update an Order: POST
/orders/{order_ref}/
- Retrieve an Order: GET
- Order Refunds
- Create a refund for an entire Order: POST
/orders/{order_ref}/refund_all/
- Create a refund for part of an Order: POST
/orders/{order_ref}/refunds
- Retrieve an OrderRefund: GET
/orders/{order_ref}/refunds/{refund_ref}/
- Create a refund for an entire Order: POST
- Payments
- Create a Payment: POST
/payments/
- Retrieve a Payment: GET
/payments/{payment_ref}/
- Update a Payment: POST
/payments/{payment_ref}/
- Create a Payment: POST
- Payment Refunds
- Create a PaymentRefund: POST
/payments/{payment_ref}/refunds/
- Retrieve a PaymentRefund for a Payment: GET
/payments/{payment_ref}/refunds/{refund_ref}/
- Create a PaymentRefund: POST
SDK methods that return a receipt
receipt
All SDK methods that capture an EBT payment return a receipt.
- Android:
capturePayment(pinForageEditText, paymentRef)
- iOS:
ForageSDK.shared.capturePayment(foragePinTextField, paymentReference)
- JS:
forage.capturePayment(ForagePinElement, paymentRef)
How to extract data from a receipt
receipt
The following receipt
fields include data that FNS requires merchants to display to EBT cardholders:
transaction_type
snap_amount
ebt_cash_amount
other_amount
sales_tax_applied
last_4
You can send a request to any of the Forage endpoints that returns a receipt
and extract the required fields from the response. The following examples fetch an Order
:
function fetchOrderDetails(orderRef = '', sessionToken = '', merchantId = '') {
const url = `https://api.sandbox.joinforage.app/api/orders/${orderRef}/`;
const headers = {
'API-Version': '2023-05-15',
'Authorization': `Bearer ${sessionToken}`,
'Merchant-Account': merchantId,
'accept': 'application/json'
};
fetch(url, {
method: 'GET',
headers: headers
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
}
curl --request GET \
--url https://api.sandbox.joinforage.app/api/orders/{order_ref}/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Merchant-Account: 9000055' \
--header 'accept: application/json' \
--header 'content-type: application/json'
Then you can display the data, as in these examples:
import React from "react";
const DataDisplay = (props) => {
const {
// From the Forage receipt object
transaction_type,
snap_amount,
ebt_cash_amount,
other_amount,
sales_tax_applied,
last_4,
// Merchant-provided or calculated
total_amount,
store_name,
contact_info,
transaction_date,
transaction_time,
snap_balance,
ebt_balance,
delivery_address,
fees
} = props
return (
<div>
<p>Store Name: {data.store_name}</p>
<p>Transaction Date: {data.transaction_date}</p>
<p>Transaction Time: {data.transaction_time}</p>
<p>Transaction Type: {data.transaction_type}</p>
<p>Snap Amount: {data.snap_amount}</p>
<p>EBT Cash Amount: {data.ebt_cash_amount}</p>
<p>Other Amount: {data.other_amount}</p>
<p>Sales Tax Applied: {data.sales_tax_applied}</p>
<p>Fees: {data.fees}</p>
<p>Order Total: {data.total_amount}</p>
<p>Remaining SNAP balance: {data.snap_balance}</p>
<p>Remaining EBT Cash balance: {data.ebt_balance}</p>
<p>Last 4 Card Digits: {data.last_4}</p>
<p>Delivery Address: {data.delivery_address}</p>
<p>Contact Info: {data.contact_info}</p>
</div>
);
};
export default DataDisplay;
import UIKit
class DataDisplayView: UIView {
var data: [String: Any] = [:] {
didSet {
updateUI()
}
}
// Example labels to display the data
let storeNameLabel = UILabel()
let transactionDateLabel = UILabel()
let transactionTimeLabel = UILabel()
let transactionTypeLabel = UILabel()
let snapAmountLabel = UILabel()
let ebtCashAmountLabel = UILabel()
let otherAmountLabel = UILabel()
let salesTaxAppliedLabel = UILabel()
let feesLabel = UILabel()
let orderTotalLabel = UILabel()
let snapBalanceLabel = UILabel()
let ebtCashBalanceLabel = UILabel()
let last4Label = UILabel()
let deliveryAddressLabel = UILabel()
let contactInfoLabel = UILabel()
override init(frame: CGRect) {
super.init(frame: frame)
setupUI()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
setupUI()
}
private func setupUI() {
addSubview(storeNameLabel)
addSubview(transactionDateLabel)
addSubview(transactionTimeLabel)
addSubview(transactionTypeLabel)
addSubview(snapAmountLabel)
addSubview(ebtCashAmountLabel)
addSubview(otherAmountLabel)
addSubview(salesTaxAppliedLabel)
addSubview(feesLabel)
addSubview(orderTotalLabel)
addSubview(snapBalanceLabel)
addSubview(ebtCashBalanceLabel)
addSubview(last4Label)
addSubview(deliveryAddressLabel)
addSubview(contactInfoLabel)
// Set layout constraints using AutoLayout or other layout methods
storeNameLabel.frame = CGRect(x: 0, y: 0, width: frame.width, height: 20)
transactionDateLabel.frame = CGRect(x: 0, y: 30, width: frame.width, height: 20)
transactionTimeLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
transactionTypeLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
snapAmountLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
ebtCashAmountLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
otherAmountLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
salesTaxAppliedLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
feesLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
orderTotalLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
snapBalanceLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
ebtCashBalanceLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
last4Label.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
deliveryAddressLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
contactInfoLabel.frame = CGRect(x: 0, y: 60, width: frame.width, height: 20)
updateUI()
}
private func updateUI() {
storeNameLabel.text = "Store Name: \(data["store_name"] ?? "")"
transactionDateLabel.text = "Transaction Date: \(data["transaction_date"] ?? "")"
transactionTimeLabel.text = "Transaction Time: \(data["transaction_time"] ?? "")"
transactionTypeLabel.text = "Transaction Type: \(data["transaction_type"] ?? "")"
snapAmountLabel.text = "Snap Amount: \(data["snap_amount"] ?? "")"
ebtCashAmountLabel.text = "EBT Cash Amount: \(data["ebt_cash_amount"] ?? "")"
otherAmountLabel.text = "Other Amount: \(data["other_amount"] ?? "")"
salesTaxApplied.text = "Sales Tax Applied: \(data["sales_tax_applied"] ?? "")"
feesLabel.text = "Fees: \(data["fees"] ?? "")"
orderTotalLabel.text = "Order Total: \(data["total_amount"] ?? "")"
snapBalanceLabel.text = "Remaining SNAP balance: \(data["snap_balance"] ?? "")"
ebtCashBalanceLabel.text = "Remaining EBT Cash balance: \(data["ebt_balance"] ?? "")"
last4Label.text = "Last 4 Card Digits: \(data["last4"] ?? "")"
deliveryAddressLabel.text = "Delivery Address: \(data["delivery_address"] ?? "")"
contactInfoLabel.text = "Contact Info: \(data["contact_info"] ?? "")"
}
}
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val data = intent.getBundleExtra("data") ?: Bundle()
updateUI(data)
}
private fun updateUI(data: Bundle) {
val storeNameTextView = findViewById<TextView>(R.id.storeNameTextView)
val transactionDateTextView = findViewById<TextView>(R.id.transactionDateTextView)
val transactionTimeTextView = findViewById<TextView>(R.id.transactionTimeTextView)
val transactionTypeTextView = findViewById<TextView>(R.id.transactionTypeTextView)
val snapAmountTextView = findViewById<TextView>(R.id.snapAmountTextView)
val ebtCashAmountTextView = findViewById<TextView>(R.id.ebtCashAmountTextView)
val otherAmountTextView = findViewById<TextView>(R.id.otherAmountTextView)
val salesTaxAppliedTextView = findViewById<TextView>(R.id.salesTaxAppliedTextView)
val feesTextView = findViewById<TextView>(R.id.feesTextView)
val orderTotalTextView = findViewById<TextView>(R.id.orderTotalTimeTextView)
val snapBalanceTextView = findViewById<TextView>(R.id.snapBalanceTextView)
val ebtCashBalanceTextView = findViewById<TextView>(R.id.ebtCashBalanceTextView)
val last4TextView = findViewById<TextView>(R.id.last4TextView)
val deliveryAddressTextView = findViewById<TextView>(R.id.deliveryAddressTextView)
val contactInfoTextView = findViewById<TextView>(R.id.contactInfoTextView)
storeNameTextView.text = "Store Name: ${data.getString("store_name")}"
transactionDateTextView.text = "Transaction Date: ${data.getString("transaction_date")}"
transactionTimeTextView.text = "Transaction Time: ${data.getString("transaction_time")}"
transactionTypeTextView.text = "Transaction Type: ${data.getString("transaction_type")}"
snapAmountTextView.text = "SNAP Amount: ${data.getString("transaction_time")}"
ebtCashAmountTextView.text = "EBT Cash Amount: ${data.getString("transaction_time")}"
otherAmountTextView.text = "Other Amount: ${data.getString("transaction_time")}"
salesTaxAppliedTextView.text = "Sales Tax: ${data.getString("transaction_time")}"
feesLabelTextView.text = "Fees: ${data.getString("transaction_time")}"
orderTotalTextView.text = "Order Total: ${data.getString("transaction_time")}"
snapBalanceTextView.text = "Remaining SNAP Balance: ${data.getString("transaction_time")}"
ebtCashBalanceTextView.text = "Remaining EBT Cash Balance: ${data.getString("transaction_time")}"
last4TextView.text = "Last 4 Card Digits: ${data.getString("transaction_time")}"
deliveryAddressTextView.text = "Delivery Address: ${data.getString("transaction_time")}"
contactInfoTextView.text = "Contact Info: ${data.getString("transaction_time")}"
}
}
Online confirmation screens and receipts
To protect EBT SNAP cardholders, FNS requires that merchants provide confirmation screens and receipts to customers following purchases or refunds that involve government benefits.
A confirmation screen must be displayed immediately after a customer completes a purchase. It documents order details and alerts a customer to the status of their transaction.
An email receipt provides customers with proof of purchase. FNS mandates that merchants either send an email receipt or add the required receipt details to a customer’s online account history within 24 hours after the order is submitted.
The following sections outline the requirements for confirmation screens and email receipts, for both EBT SNAP purchases and refunds.
EBT purchases
Confirmation screen requirements for EBT purchases
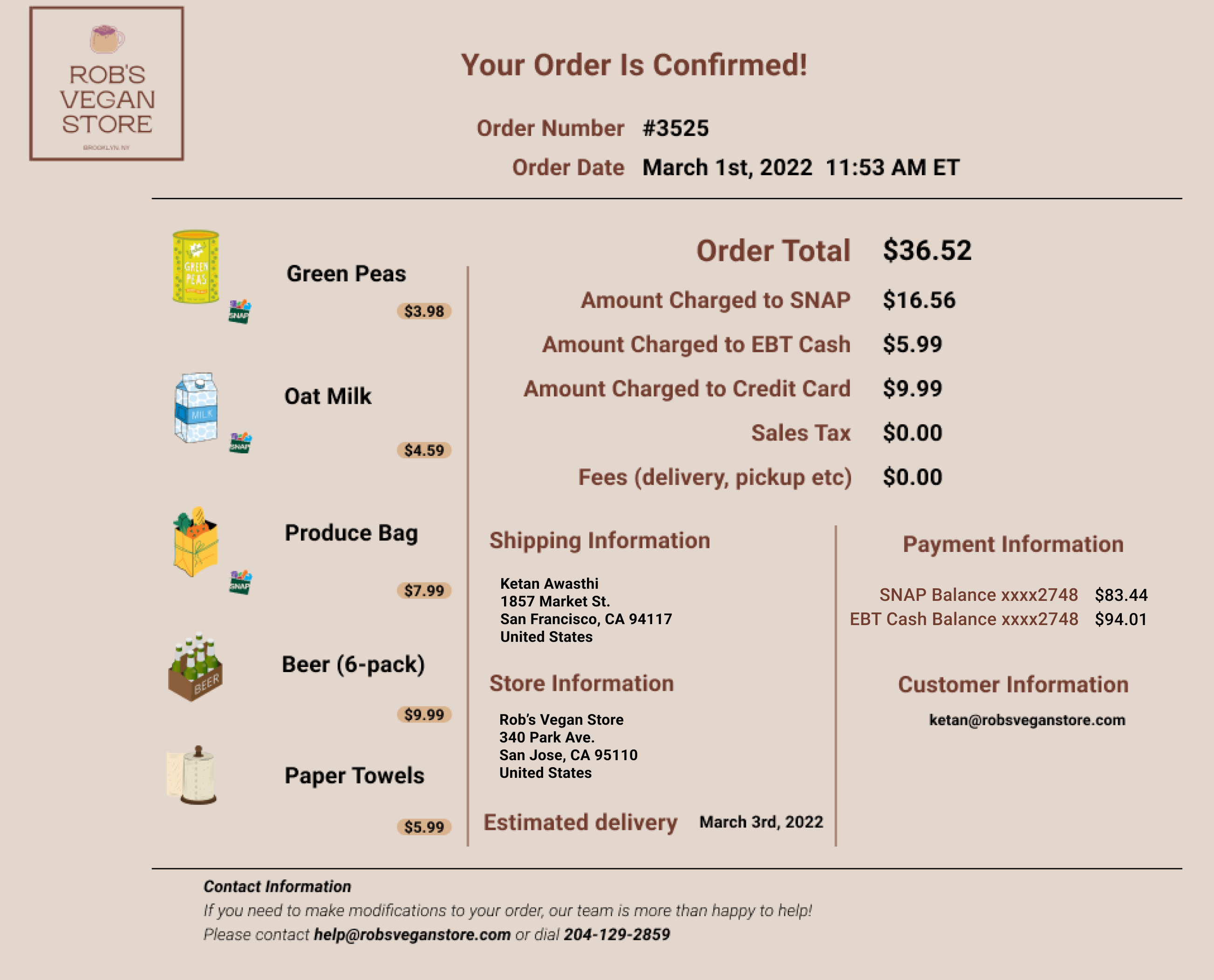
Example purchase confirmation screen
Requirement | Where to find the data | Example value |
---|---|---|
Merchant name | Merchant-provided | Rob’s Vegan Store |
Merchant contact information* *It’s not required to provide a physical address if non-EBT receipts do not currently display a physical address. | Merchant-provided | Contact Information If you need to make modifications to your order, our team is more than happy to help! Please contact [email protected] or dial 204-129-2859 |
Transaction date | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the Session in periodic GET requests to /orders/{order_ref}/ until the success_date field populates.SDKs:- Pass the ref returned in the response to the request that created the Payment in periodic GET requests to /payments/{payment_ref}/ until the success_date field populates. | Order Date March 1st, 2022 11:53 AM ET |
Transaction time_ _A timestamp is only required if non-EBT receipts currently display a timestamp.* | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the Session in periodic GET requests to /orders/{order_ref}/ until the success_date field populates.SDKs:- Pass the ref returned in the response to the request that created the Payment in periodic GET requests to /payments/{payment_ref}/ until the success_date field populates. | Order Date March 1st, 2022 11:53 AM ET |
Transaction type | receipt.transaction_type | Your Order Is Confirmed! |
Truncated EBT Card Number | receipt.last_4 | xxxx2748 |
Total transacted amount | Sum the following receipt fields to calculate the total cost:snap_amount + ebt_cash_amount + other_amount + sales_tax_applied | $36.52 |
Tender type(s) and amount(s) | - receipt.snap_amount - receipt.ebt_cash_amount - receipt.other_amount - receipt.sales_tax_applied | Amount Charged to SNAP $16.56 Amount Charged to EBT Cash $5.99 Amount Charged to Credit Card $9.99 Sales Tax $0.00 |
Remaining SNAP and/or EBT Cash balance | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the Session in periodic GET requests to /orders/{order_ref}/ until the status of the Order is succeeded . At that point, the receipt.balance is up-to-date.SDKs:- Pass the ref returned in the response to the request that created the Payment in periodic GET requests to /payments/{payment_ref}/ until the status of the Payment is succeeded . At that point, the receipt.balance is up-to-date. | SNAP Balance xxxx2748 $83.44 EBT Cash Balance xxxx2748 $94.01 |
Delivery or pickup address | Either the delivery_address field of the response object of the request that created the Session (Fully Hosted & Custom) or Payment (SDKs), or merchant-provided. | Ketan Awasthi 1857 Market St. San Francisco, CA 94117 United States |
Scheduled delivery or pickup date | Merchant-provided | Estimated delivery March 3rd, 2022 |
Scheduled delivery or pickup time, if applicable | Merchant-provided | N/A |
Itemized fees for delivery/pickup/shipping, ordering, convenience, handling or other fees or charges | Merchant-provided | Fees (delivery, pickup, etc.) $0.00 |
Itemized fees for bags or other delivery/pickup/shipping containers | Merchant-provided | Fees (delivery, pickup, etc.) $0.00 |
Email receipt requirements for EBT purchases
Receipt Delivery Alternatives
Instead of emailing receipts, you may provide a password-protected account interface for customers to view their transaction history.
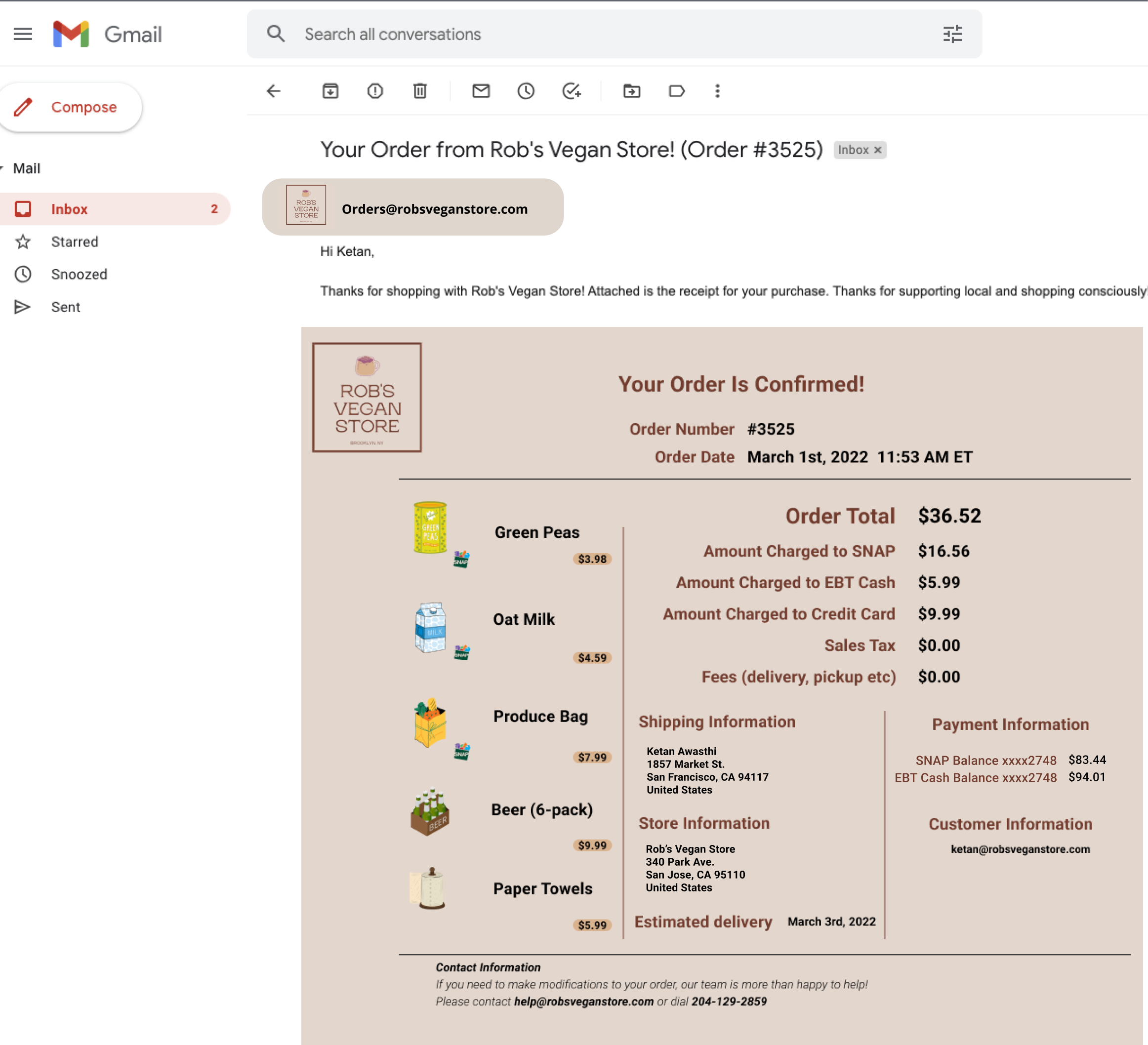
Example purchase receipt
Requirement | Where to find the data | Example value |
---|---|---|
Transaction date and time | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the Session in periodic GET requests to /orders/{order_ref}/ until the success_date field populates.SDKs:- Pass the ref returned in the response to the request that created the Payment in periodic GET requests to /payments/{payment_ref}/ until the success_date field populates. | Order Date March 1st, 2022 11:53 AM ET |
Merchant name | Merchant-provided | Rob’s Vegan Store |
Merchant contact information | Merchant-provided | Contact Information If you need to make modifications to your order, our team is more than happy to help! Please contact [email protected] or dial 204-129-2859 |
Delivery or pickup address | Either the delivery_address field of the response object of the request that created the Session (Fully Hosted & Custom) or Payment (SDKs), or merchant-provided. | Ketan Awasthi 1857 Market St. San Francisco, CA 94117 United States |
Scheduled delivery or pickup time | Merchant-provided | March 3rd, 2022 |
Transaction program | Merchant-provided, or parse the purchase receipt fields for where the value is not zero for snap_amount or ebt_cash_amount . | Amount Charged to SNAP Amount Charged to EBT Cash |
Tender type(s) and amount(s) | - receipt.snap_amount - receipt.ebt_cash_amount - receipt.other_amount - receipt.sales_tax_applied | Amount Charged to SNAP $16.56 Amount Charged to EBT Cash: $5.99 Amount Charged to Credit Card $9.99 Sales Tax $0.00 |
Total transacted amount | Add the following receipt fields to calculate the total cost:snap_amount + ebt_cash_amount + other_amount + sales_tax_applied | $36.52 |
Transaction type | receipt.transaction_type | Your Order Is Confirmed! |
Remaining SNAP and/or EBT Cash balance | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the Session in periodic GET requests to /orders/{order_ref}/ until the status of the Order is succeeded. At that point, the receipt.balance.snap data is up-to-date.SDKs:- Pass the ref returned in the response to the request that created the Payment in periodic GET requests to /payments/{payment_ref}/ until the status of the Payment is succeeded . At that point, the receipt.balance.snap is up-to-date. | SNAP Balance xxxx2748 $83.44 EBT Cash Balance xxxx2748 $94.01 |
Truncated EBT Card Number | receipt.last_4 | xxxx2748 |
While not required by FNS, Forage also recommends that merchants display the following information on receipts or order history pages:
Recommendation | Where to find the data | Example value |
---|---|---|
Transaction number | Either the Forage-provided ref for the Order (Fully Hosted & Custom) or Payment (SDKs), or merchant-provided. | Order Number #3525 |
Item details | Fully Hosted: - Either the product_list property of the Session object returned when the Session was created, or merchant-provided.Custom:- Merchant-providedSDKs: - Merchant-provided | Green Peas 3.98 Oat Milk $4.59 Produce Bag $7.99 Beer (6-Pack) $9.99 Paper Towels $5.99 |
Itemized fees for delivery/pickup/shipping, ordering, convenience, handling or other fees or charges | Merchant-provided | Fees (delivery, pickup etc) $0.00 |
Fees (delivery, pickup etc) $0.00 | Merchant-provided | Fees (delivery, pickup etc) $0.00 |
EBT refunds
In the case of EBT SNAP refunds, FNS requires that merchants notify customers. The notification can be either a confirmation screen or an email receipt. You are welcome to provide customers with both, but only one is mandatory.
It’s worth highlighting a few specific scenarios:
- If an EBT refund is processed before an order number is successfully generated, then you are not required to provide a receipt or order confirmation screen. This can happen if there’s an unsuccessful charge to a secondary payment method, or if there’s insufficient inventory to fulfill a purchase.
- If you display an itemized list of refund details in a customer’s account transaction history, then you’re not required to list itemized details on the refund confirmation screen or email receipt.
- There is no requirement for an item’s price to be displayed on the refund confirmation screen, email receipt, or in the customer’s account transaction history.
Information requirements for EBT refund notifications
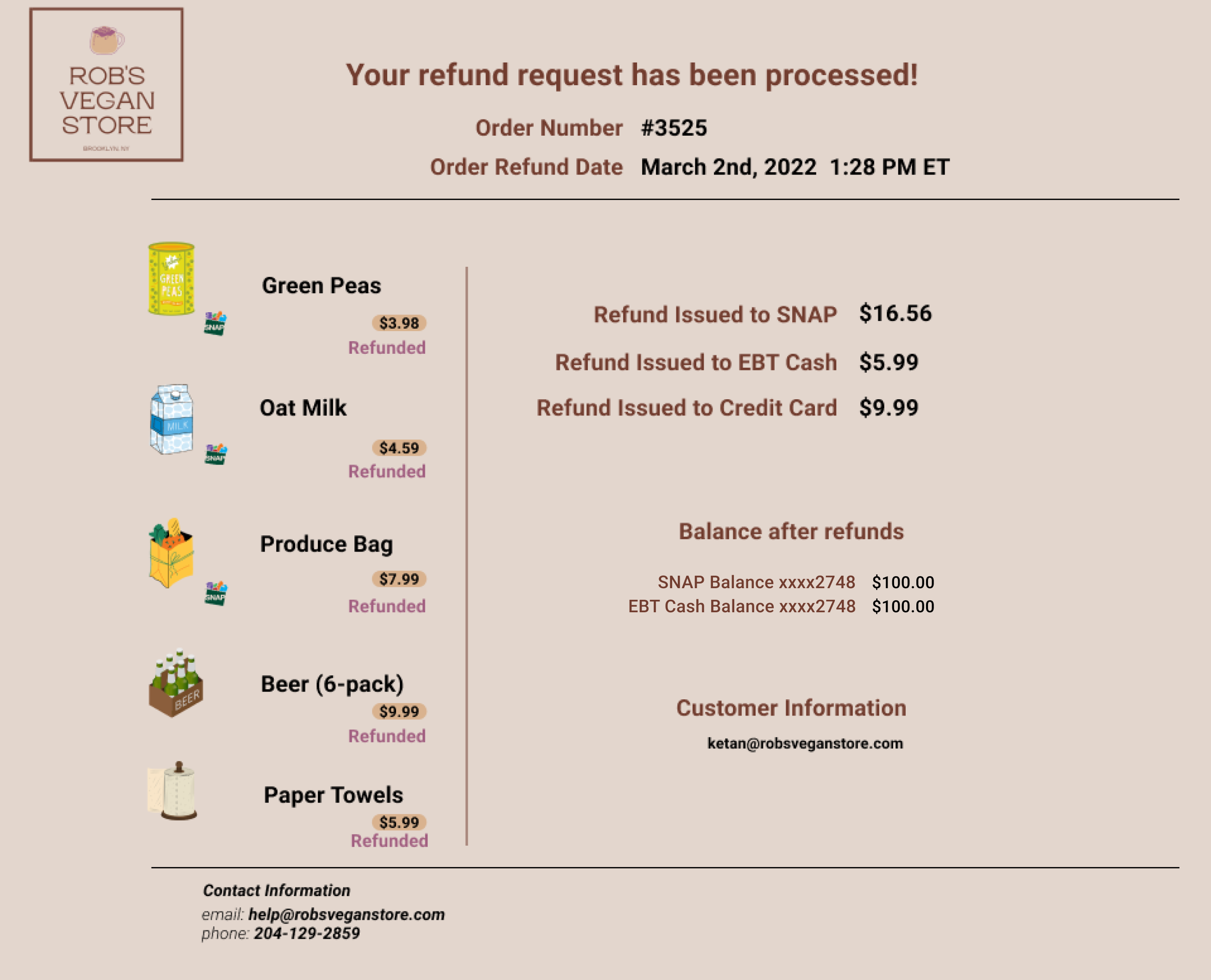
Example refund confirmation screen
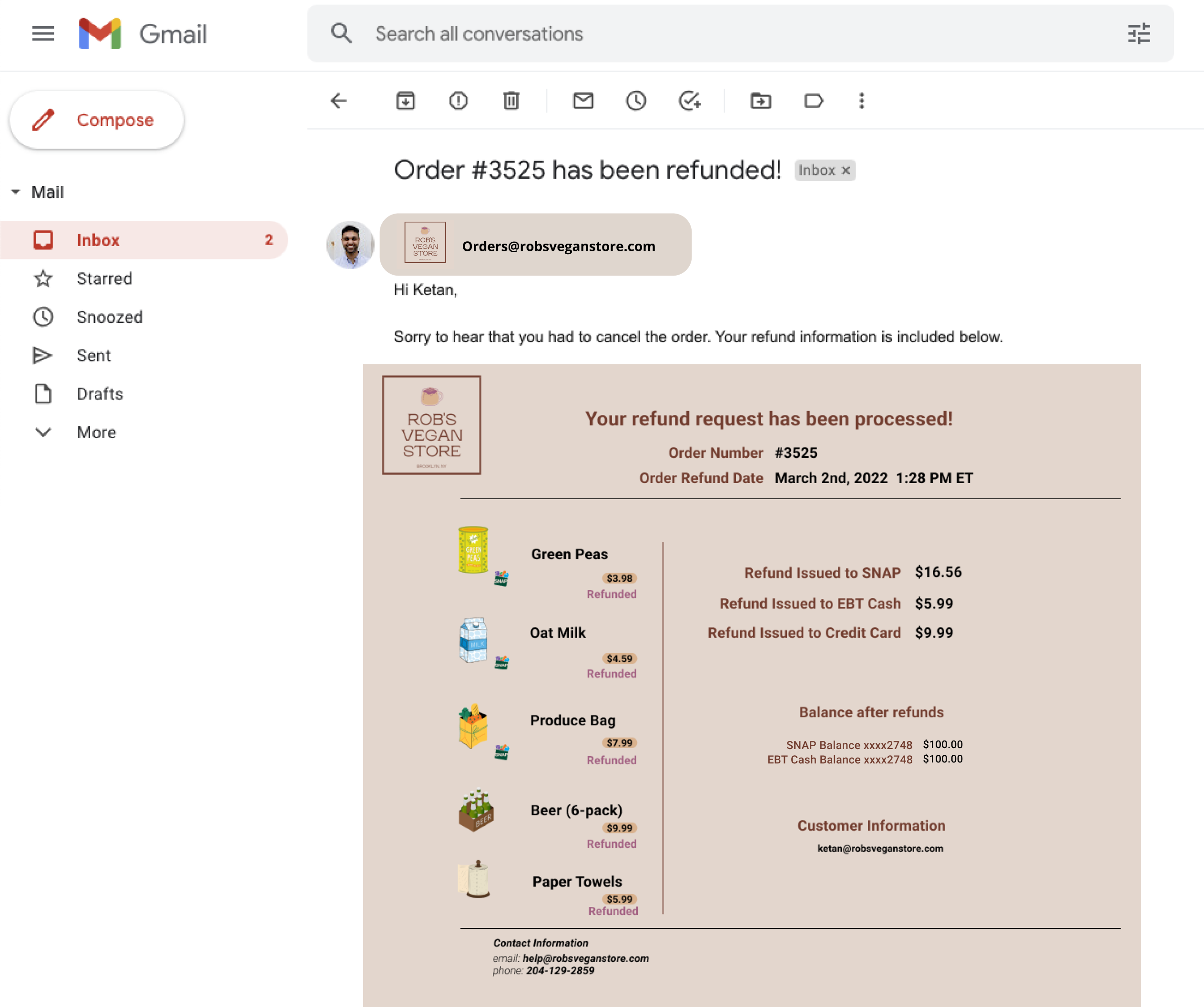
Example refund email receipt
Requirement | Where to find the data | Example value |
---|---|---|
Transaction date and time | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the Session in periodic GET requests to /orders/{order_ref}/ until the success_date field populates.SDKs:- Pass the ref returned in the response to the request that created the Payment in periodic GET requests to /payments/{payment_ref}/ until the success_date field populates. | Order Date March 1st, 2022 11:53 AM ET |
Merchant name | Merchant-provided | Rob’s Vegan Store |
Merchant contact information | Merchant-provided | Contact Information email: [email protected] phone: 204-129-2859 |
Transaction program | Merchant-provided, or parse the refund receipt fields for where the value is not zero for snap_amount , ebt_cash_amount , other_amount or sales_tax_applied . | Refund Issued to SNAP Refund Issued to EBT Cash Refund Issued to Credit Card |
Tender type(s) and amount(s) credited for the refund | - receipt.snap_amount - receipt.ebt_cash_amount - receipt.other_amount - receipt.sales_tax_applied | Refund Issued to SNAP $16.56 Refund Issued to EBT Cash $5.99 Refund Issued to Credit Card $9.99 |
Remaining SNAP and/or EBT Cash balance | Fully Hosted & Custom: - Pass the ref returned in the response to the request that created the OrderRefund in periodic GET requests to /orders/{order_ref}/refunds/{refund_ref}/ until the status of the OrderRefund is succeeded . At that point, the receipt.balance is up-to-date.SDKs:- Pass the ref returned in the response to the request that created the PaymentRefund in periodic GET requests to /payments/{payment_ref}/refunds/{refund_ref}/ until the status of the PaymentRefund is succeeded . At that point, the receipt.balance is up-to-date. | SNAP Balance xxxx2748 $100.00 EBT Cash Balance xxxx2748 $100.00 |
Truncated EBT Card Number | receipt.last_4 | xxxx2748 |
While not required by FNS, Forage also recommends that merchants display the following information on receipts or order history pages:
Recommendation | Where to find the data | Example value |
---|---|---|
Original transaction number | Either the Forage-provided ref for the OrderRefund (Fully Hosted & Custom) or PaymentRefund (SDKs), or merchant-provided. | Order Number #3525 |
Transaction type | receipt.transaction_type | Your refund request has been processed! |
POS Terminal receipts
Terminal integrations.
This section is specific to Forage Terminal. For details, see the guide to building a Forage POS integration.
For EBT SNAP payments and refunds processed through POS terminals, FNS requires merchants to offer customers the option to receive a printed receipt during the transaction.
FNS mandates that every receipt displays a specific set of information to protect cardholders.
The example receipts and corresponding table below outline these FNS requirements.
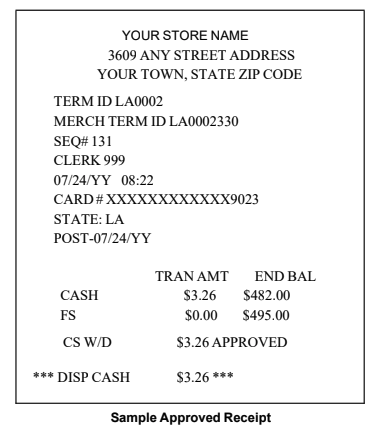
Sample Approved Receipt
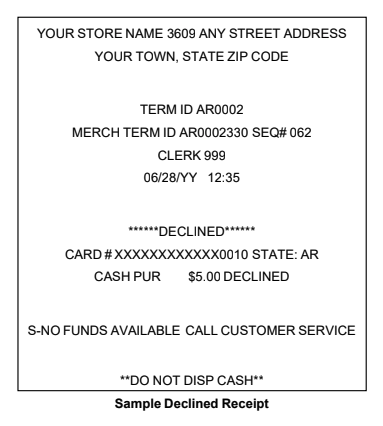
Sample Declined Receipt
Requirement | Where to find the data | Example Value |
---|---|---|
Transaction date | The timestamp must reflect when the customer initiated the transaction. Find this data on receipt.created . | 07/24/YY |
Merchant name and location | Merchant-provided | YOUR STORE NAME 36069 ANY STREET ADDRESS YOUR TOWN, STATE ZIP CODE |
Transaction type | receipt.transaction_type The exact Forage value is either Payment or Refund . The printed receipt that you provide the customer should reflect this transaction type. | CASH PUR |
Tender type(s) and amount(s) | - receipt.snap_amount - receipt.ebt_cash_amount | TRAN AMT CASH $3.26 |
Remaining SNAP and/or EBT Cash balance | One of: - Send periodic GET requests to /payments/{payment_ref}/ until the status of the Payment is succeeded. At that point, the receipt.balance is up-to-date.- Call the checkBalance SDK method | END BAL $482.00 |
Truncated EBT Card Number | receipt.last_4 | CARD # XXXXXXXXXXXX9023 |
Terminal location* *The Terminal location can be a street address, generally accepted name for the specific location, or the name of the owner or operator of the terminal. | Merchant-provided | TERM ID LA0002 MERCH TERM ID LA0002330 SEQ# 131 CLERK 999 |
Transaction status: Approved or Denied* *Denied transactions also require a reason for the denial to be displayed on the receipt. | Pass the ref returned in the response to create the Payment in periodic GET requests to /payments/{payment_ref}/ to retrieve the payment.status .If the status is succeeded , then the transaction has been approved.If the status is failed , then the transaction has been denied. The reason for the decline can be found in the receipt.message field of the response. | Receipt #1: APPROVED Receipt #2: DENIED ... S-NO FUNDS AVAILABLE CALL CUSTOMER SERVICE |
Transaction number | Either the Forage-provided ref for the Payment , or merchant-provided. | SEQ# 131 |
POS Refund Receipts
A refund receipt must match the original transaction, except for the negative amount and updated EBT card balance.
While not required by FNS, Forage also recommends that merchants display the following information on POS receipts:
Recommendation | Where to find the data | Example value |
---|---|---|
Item details | Merchant-provided | N/A |
Itemized fees, if applicable, for delivery/pickup/shipping, ordering, convenience, handling, or other fees or charges | Merchant-provided | N/A |
Transaction time | The timestamp must reflect when the customer initiated the transaction. Find this data on receipt.created . | 08:22 |
Updated about 1 month ago