JS
Add EBT payments to your web application
You can use the Forage JS SDK to process EBT payments in your web application.
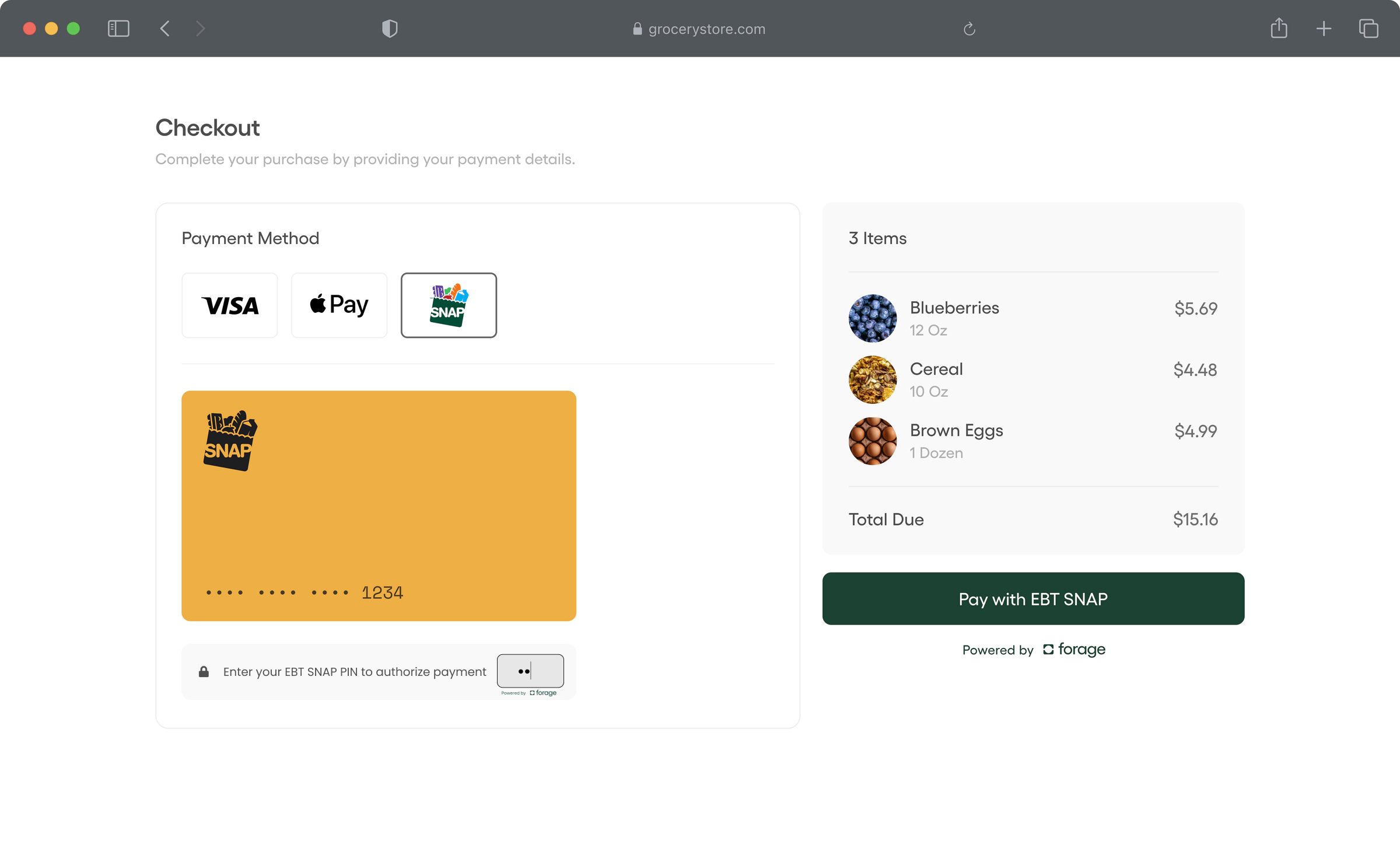
Features
- Native UI component for collecting and tokenizing a customer’s EBT Card number (also called the card PAN)
- Native UI component for securely collecting a customer’s EBT PIN
- Built-in EBT Card number validation
- Control over the styling of a native EBT checkout experience
- Methods that securely collect and tokenize an EBT Card number, perform a balance check, and capture a payment
Requirements
- Before you can use the Forage JS SDK, make sure that you have:
- Met all of the prerequisites for working with Forage
- Signed up for an account. Contact us if you don’t yet have one.
- Set up your development environment
Starter code and EBT Element life cycle
For comprehensive instructions, refer to the complete Forage JS documentation.
The EBT Element lifecycle contains the following steps:
- Initialize
- Create
- Attach event listeners
- Mount
- Submit
- Destroy
Step 1: Initialize
const forage = Forage({
merchantId: '123ab45c67',
sessionToken:
'sandbox_eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJhIjo0MiwiZXhwIjoxNjc2OTg2ODM1fQ.7KLvDpM9WHRD4pKCYr0rOy2kM0hVf3bwtZEAOZJrJ1w',
// NOTE: The following line is for testing purposes only and should not be used in production.
// Please replace this line with a real hashed customer ID value.
customerId: btoa(Math.random().toString()),
appearance: {
variables: {
borderRadius: '8px'
}
}
})
Refer to the Initialize Forage JS docs for complete documentation.
Step 2: Create an EBT Element
To create an EBT Element, invoke the create()
method on the instance of the Forage object, passing in the usecase
that corresponds to the target element type.
The following example creates a ForageCardElement
that a customer can use to securely input their EBT Card number:
const tokenizeCardElement = forage.create('tokenize_card')
Step 3: Attach event listeners
The following example displays a message if there's an event.error
on change
:
tokenizeCardElement.on('change', (event) => {
const explainErrorSpan = document.getElementById('explain-error')
if (event.error) {
// show validation error to customer
explainErrorSpan.textContent = event.error.message
} else {
// hide validation error
explainErrorSpan.textContent = ''
}
})
Step 4: Mount the EBT Element
Mount the element to the DOM:
tokenizeCardElement.mount('card-entry-container')
Step 5: Submit the EBT Element
Call the EBT Element's submit()
method to securely transmit the customer input to Forage, as in the example below:
try {
const tokenizationResult = await forage.tokenizeCard(
tokenizeCardElement
)
const { ref: paymentMethodRef, type, balance, card } = tokenizationResult
} catch (error) {
const { httpStatusCode, message, code } = error ?? {}
}
Step 6: Destroy the EBT Element
tokenizeCardElement.destroy()
Next steps
- Explore Forage JS reference docs
Updated 6 months ago