An OrderRefund
represents a refund associated with an existing Order
object
OrderRefund
objects are only relevant to Fully Hosted and Custom integrations.If you’re building with a Forage SDK, then use
PaymentRefunds
.
The Forage API /orders/{order_ref}/refunds/
endpoints process refunds to an existing Order
. With the Forage Payments API, you can:
- Create a refund for an entire Order: POST
/orders/{order_ref}/refund_all/
- Create a refund for part of an Order: POST
/orders/{order_ref}/refunds/
- Retrieve all OrderRefunds for an Order: GET
/orders/{order_ref}/refunds/
- Retrieve an OrderRefund: GET
/orders/{order_ref}/refunds/{refund_ref}/
You need to pass an order_ref
for an existing Order
object as a path param to create or retrieve an OrderRefund
. The order_ref
is the ref
value returned in the response to the original request to create a Forage Session.
OrderRefund
object
OrderRefund
objectA request to create a partial OrderRefund returns a single OrderRefund
object. A request to refund an entire order returns the original Forage Order
object. The refunds
field of the Order
details a list of reference hashes for all OrderRefunds
that Forage created as part of the complete refund.
OrderRefund
objects take the following shape:
{
"ref": "1ccabfg754",
"order": "e0f7607d5f",
"payment": "f587edf124",
"merchant": "9000055",
"funding_type": "ebt_snap",
"amount": "20.00",
"merchant_fixed_settlement": 5.95,
"platform_fixed_settlement": 5.11,
"reason": "Order could not be delivered.",
"metadata": {},
"created": "2023-09-18T11:38:29.279185-07:00",
"updated": "2023-09-18T11:38:29.279209-07:00",
"status": "requires_confirmation",
"last_processing_error": null,
"receipt": null,
"tpp_lookup_id": "re_3JemUSGfBYJeLEva0af6My64",
"refund_errors": []
}
Properties
Property | Type | Description |
---|---|---|
ref | string | A unique reference hash for the OrderRefund . |
order | string | The unique reference hash for the parent Forage Order . |
payment | string | The unique reference hash for the OrderPayment to be refunded. |
merchant | string | A string that represents either the merchant’s FNS number, or a unique merchant ID that Forage provides during onboarding. |
funding_type | string | A string that represents the type of tender. One of: - benefit - credit_tpp - ebt_snap - ebt_cash |
amount | number | A positive decimal number that represents how much of the OrderPayment to refund in USD. If a partial refund, then this value does not need to equal the amount field of the original OrderPayment . Precision to the penny is supported.The minimum amount that can be refunded is 0.01 . |
merchant_fixed_settlement | number | The fixed amount in USD that should be restored to the merchant from EBT Cash payments prior to splitting by the platform_fee . Precision to the penny is supported. |
platform_fixed_settlement | number | The fixed amount in USD that should be restored to the platform from EBT Cash payments prior to splitting by the platform_fee . Precision to the penny is supported. |
reason | string | A string that describes why the Order is to be refunded. |
metadata | object | A set of optional, merchant-defined key-value pairs that can be added to the request data. For example, some merchants attach their credit card processor’s ID for the customer making the payment or refund. Some merchants opt to pass an empty object if they’ve got no additional information to add. |
created | ISO 8601 date-time string | A UTC-8 timestamp of when the OrderRefund was created. |
updated | ISO 8601 date-time string | A UTC-8 timestamp of when the OrderRefund was last updated. |
status | string | The status of the OrderRefund . One of:- canceled : The OrderRefund has been voided and can't be changed.- failed : The OrderRefund failed to process. Check the receipt.message field for a description of the error.- processing : The outcome of the OrderRefund is pending, and the refund can't be modified.- succeeded : The OrderRefund has been successfully processed and will be included in settlement. |
last_processing_error | object | The code and message values corresponding to the most recent Payments API error. |
receipt | object | The information that you’re required to display to the customer after the OrderRefund finishes processing, according to FNS regulations.Refer to receipt for details. |
tpp_lookup_id | string | The unique identifier from the relevant credit TPP or null for EBT refunds. For Stripe integrations, this is the id of the Refund object on Stripe's backend. |
refund_errors | array | An array of objects that details information about any refund errors. Refer to refund_errors .Note that this field is only returned when an OrderRefund is created. The retrieve an OrderRefund request omits this field in its response. |
receipt
receipt
This field is
null
when theOrderRefund
is first created and until receipt data is available. Send periodic GET requests to/orders/{order_ref}/refunds/{refund_ref}/
until you have retrieved thereceipt
data, which includes the updatedbalance
.
Property | Type | Description |
---|---|---|
ref_number | string | A unique reference hash for the Forage OrderRefund object. |
is_voided | boolean | Whether the OrderRefund has been voided. If false , then the order has been refunded as expected. In the rare case of true , the charge has been reinstated. |
snap_amount | string | The USD amount refunded to the SNAP balance of the EBT Card, represented as a numeric string. |
ebt_cash_amount | string | The USD amount refunded to the EBT Cash balance of the EBT Card, represented as a numeric string. |
other_amount | string | The USD amount refunded to any payment method that is not an EBT Card, represented as a numeric string. |
sales_tax_applied | string | The USD amount of taxes refunded to the customer’s non-EBT payment instrument, represented as a numeric string. |
balance | object | Refer to balance for details. |
last_4 | string | The last four digits of the EBT Card number. |
message | string | A message from the EBT payment network that must be displayed to the cardholder. |
transaction_type | string | A constant string that is used to identify the transaction type associated with the receipt. Always Refund in the case of order refunds. |
created | ISO 8601 date-time string | A UTC-8 timestamp of when the OrderRefund was created. |
balance
balance
Property | Type | Description |
---|---|---|
snap | string | The available SNAP balance in USD to the penny on the customer’s EBT Card, represented as a numeric string. |
non_snap | string | The available EBT Cash balance in USD to the penny on the EBT Card, represented as a numeric string. |
updated | ISO 8601 date-time string | A UTC-8 timestamp of when the funds in the account last changed. |
refund_errors
refund_errors
Every object in the refund_errors
array includes the following fields:
Property | Type | Description |
---|---|---|
path | string | The requested endpoint. |
errors | array | An array of objects that details information about the error. Refer to errors . |
errors
errors
Property | Type | Description |
---|---|---|
code | string | A short string that represents the error. |
message | string | A developer-facing message with more details about the error, not to be displayed to customers. |
source | object | An object that contains the following fields: - resource : A string that represents the Forage resource involved in the error.- ref : If applicable, the ten character reference hash of the Forage resource that caused the error. An empty string if no specific individual resource was involved. |
OrderRefund
lifecycle
OrderRefund
lifecycle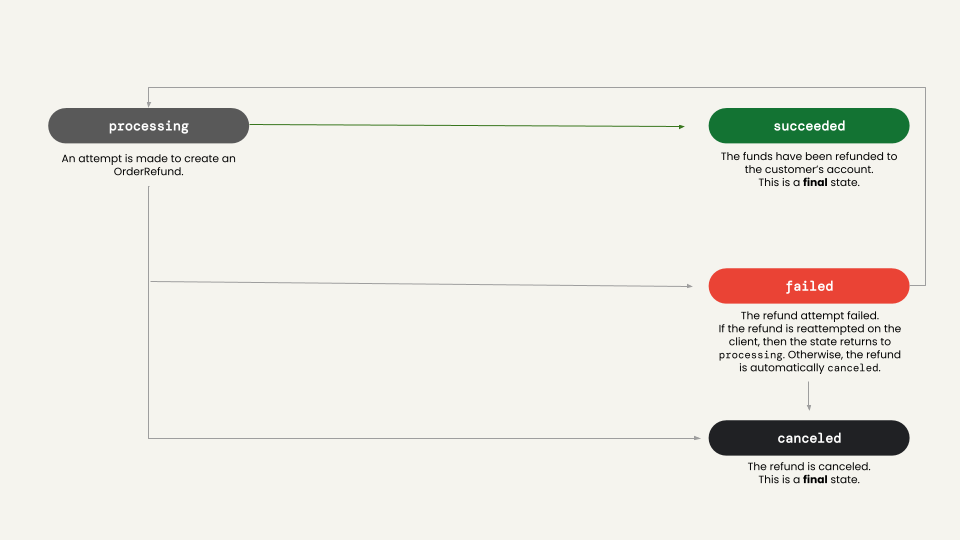
Example requests
Create an OrderRefund
for an entire Order
OrderRefund
for an entire Order
curl --request POST \
--url https://api.sandbox.joinforage.app/api/orders/order_ref/refund_all/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Idempotency-Key: 123e4567e8' \
--header 'Merchant-Account: <fns-number>' \
--header 'accept: application/json' \
--header 'content-type: application/json'
--data '
{
"reason": "Order could not be delivered",
"metadata": {}
}
'
Create a partial OrderRefund
OrderRefund
curl --request POST \
--url https://api.sandbox.joinforage.app/api/orders/order_ref/refunds/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Idempotency-Key: 123e4567e8' \
--header 'Merchant-Account: <fns-number>' \
--header 'accept: application/json' \
--header 'content-type: application/json'
--data '
{
"amount": 25.99,
"payment": <payment-ref>,
"reason": "Order could not be delivered",
"metadata": {}
}
'
Retrieve all OrderRefunds
for an Order
OrderRefunds
for an Order
curl --request GET \
--url https://api.sandbox.joinforage.app/api/orders/order_ref/refunds/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Idempotency-Key: 123e4567e8' \
--header 'Merchant-Account: <fns-number>' \
--header 'accept: application/json' \
--header 'content-type: application/json'
Retrieve an OrderRefund
OrderRefund
curl --request GET \
--url https://api.sandbox.joinforage.app/api/orders/order_ref/refunds/refund_ref/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Idempotency-Key: 123e4567e8' \
--header 'Merchant-Account: <fns-number>' \
--header 'accept: application/json' \
--header 'content-type: application/json'