A PaymentRefund
represents a refund of an existing Payment
object.
PaymentRefund
objects are only relevant to SDK integrations.If you’re building with the Fully Hosted or Custom Forage Checkout UI, then use the
OrderRefund
endpoints.
The Forage API processes refunds to an existing Payment
via PaymentRefund
objects. With the Forage Payments API, you can:
- Create a PaymentRefund: POST
/payments/{payment_ref}/refunds/
- Retrieve a PaymentRefund for a Payment: GET
/payments/{payment_ref}/refunds/{refund_ref}/
- Retrieve all PaymentRefunds for a Payment: GET
/payments/{payment_ref}/refunds/
You need to pass a payment_ref
for an existing Payment
object as a path param to create or retrieve PaymentRefunds
. The payment_ref
is the ref
value in the response object of the original request to Create a Payment.
PaymentRefund
object
PaymentRefund
object{
"ref": "0ddbcda871",
"payment_ref": "b873fe62dc",
"merchant": "9000055",
"funding_type": "ebt_snap",
"amount": "20.00",
"merchant_fixed_settlement": 5.95,
"platform_fixed_settlement": 5.11,
"reason": "Order could not be delivered.",
"metadata": {
"psp_customer_id": "cus_1234567890"
},
"created": "2023-09-18T11:38:29.279185-07:00",
"updated": "2023-09-18T11:38:29.279209-07:00",
"status": "requires_confirmation",
"last_processing_error": null,
"receipt": null
}
Properties
Property | Type | Description |
---|---|---|
ref | string | A unique reference hash for the PaymentRefund . |
payment_ref | string | The unique reference hash for the Payment to be refunded, as passed in the path params of the request. |
merchant | string | A string that represents either the merchant’s FNS number, or a unique merchant ID that Forage provides during onboarding. |
funding_type | string | A string that represents the type of tender. One of: - benefit - credit_tpp - ebt_cash - ebt_snap |
amount | number | A positive decimal number that represents how much of the Payment to refund in USD. If a partial refund, then this value does not need to equal the amount field of the original Payment . Precision to the penny is supported.The minimum amount that can be refunded is 0.01 . |
merchant_fixed_settlement | number | The fixed amount in USD that should be restored to the merchant from EBT Cash payments prior to splitting by the platform_fee . Precision to the penny is supported. |
platform_fixed_settlement | number | The fixed amount in USD that should be restored to the platform from EBT Cash payments prior to splitting by the platform_fee . Precision to the penny is supported. |
reason | string | A string that describes why the Payment is to be refunded. |
metadata | object | A set of optional, merchant-defined key-value pairs that can be added to the request data. For example, some merchants attach their credit card processor’s ID for the customer making the payment or refund. If there’s no additional information that you’d like to add, then pass an empty object as the value. |
created | ISO 8601 date-time string | A UTC-8 timestamp of when the PaymentRefund was created. |
updated | ISO 8601 date-time string | A UTC-8 timestamp of when the PaymentRefund was last updated. |
status | string | The status of the PaymentRefund . One of:- canceled : The PaymentRefund object can't be used.- failed : Check the receipt.message field for a description of the error.- processing : The outcome of the PaymentRefund is pending.- succeeded : The PaymentRefund has been successfully processed and will be included in settlement. It can't be changed. |
last_processing_error | object | The code and message values corresponding to the most recent Payments API error. |
receipt | object | The information that you’re required to display to the customer after the PaymentRefund finishes processing, according to FNS regulations.Refer to receipt for details. |
previous_errors | array | An array of objects that detail information about any errors triggered by the request. If the request was successful and without errors, then the field is returned as an empty array. Refer to previous_errors for details. |
receipt
receipt
The
receipt
value isnull
when thePaymentRefund
is first created and until receipt data is available. Pass theref
returned in the response to create thePaymentRefund
in periodic GET requests to/payments/{payment_ref}/refunds/{refund_ref}/
until thestatus
of thePaymentRefund
issucceeded
. At that point, thereceipt
data, including thebalance
, is up-to-date.
Property | Type | Description |
---|---|---|
ref_number | string | A unique reference hash for the Forage PaymentRefund object. |
is_voided | boolean | Whether the PaymentRefund has been voided. If false , then the original charge has been refunded as expected. In the rare case of true , the charge has been reinstated. |
snap_amount | string | The USD amount refunded to the SNAP balance of the EBT Card, represented as a numeric string. |
ebt_cash_amount | string | The USD amount refunded to the EBT Cash balance of the EBT Card, represented as a numeric string. |
other_amount | string | The USD amount refunded to any payment method that is not an EBT Card, represented as a numeric string. |
sales_tax_applied | string | The USD amount of taxes refunded to the customer’s non-EBT payment instrument, represented as a numeric string. |
balance | object | Refer to balance for details. |
last_4 | string | The last four digits of the EBT Card number. |
message | string | A message from the EBT payment network that must be displayed to the customer. |
transaction_type | string | A constant string that identifies the kind of transaction associated with this receipt. Always Refund for PaymentRefunds . |
created | ISO 8601 date-time string | A UTC-8 timestamp of when the PaymentRefund was created. |
balance
balance
Property | Type | Description |
---|---|---|
snap | string | The available SNAP balance in USD to the penny on the customer’s EBT Card, represented as a numeric string. |
non_snap | string | The available EBT Cash balance in USD to the penny on the EBT Card, represented as a numeric string. |
updated | ISO 8601 date-time string | A UTC-8 timestamp of when the funds in the account last changed. |
previous_errors
previous_errors
Each object in the previous_errors
array includes the following fields:
Property | Type | Description |
---|---|---|
code | string | A short string that represents the error. |
message | string | A developer-facing message with more details about the error, not to be displayed to customers. |
source | object | An object that details the specific source of the Forage error, including two keys: - resource : The type of the Forage resource involved in the error.- ref : If applicable, the ten character reference hash of the Forage resource that caused the error. An empty string if no specific individual resource was involved. |
details | object | Additional details about the error, if applicable. |
PaymentRefund
lifecycle
PaymentRefund
lifecycle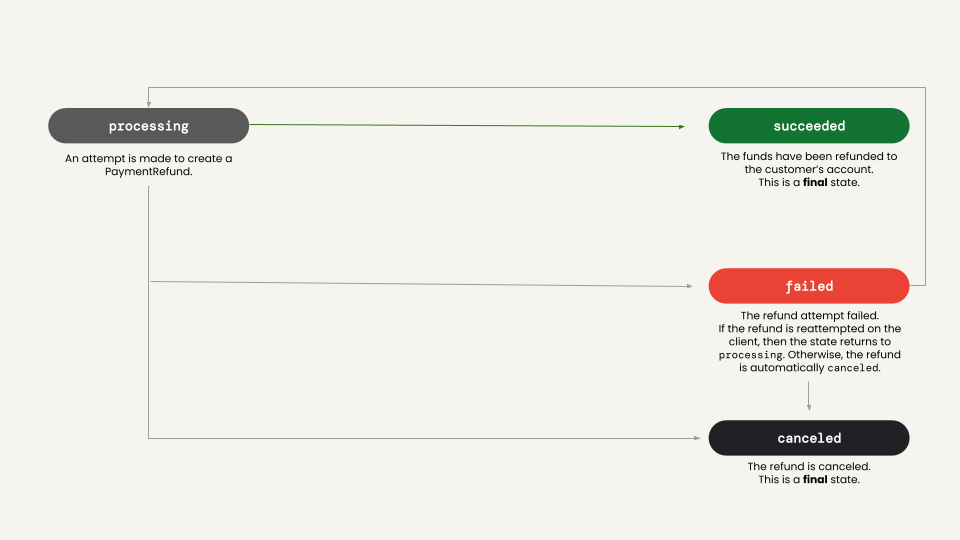
Example requests
Create a PaymentRefund
PaymentRefund
curl --request POST \
--url https://api.sandbox.joinforage.app/api/payments/<payment-ref>/refunds/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Idempotency-Key: <idempotency-key>' \
--header 'Merchant-Account: <merchant-account>' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"amount": 25.99,
"reason": "Order could not be delivered"
}
'
Retrieve all PaymentRefunds
for a Payment
PaymentRefunds
for a Payment
curl --request GET \
--url https://api.sandbox.joinforage.app/api/payments/payment_ref/refunds/ \
--header 'Authorization: Bearer <authentication-token>' \
--header 'Idempotency-Key: 123e4567e8' \
--header 'Merchant-Account: <fns-number>' \
--header 'accept: application/json' \
--header 'content-type: application/json'